Syntax
[Axis/Group] VAR32D = VAR32S
|
[A/G] long VAR32D = local VAR32S
|
[Axis/Group] VAR32D, DM = VAR32S
|
[A/G] long VAR32D, DM = local VAR32S
|
[Axis/Group] (VAR16D), TypeMem = VAR32S
|
[A/G] &(VAR16D), TM = local VAR32S
|
[Axis/Group] (VAR16D+), TypeMem = VAR32S
|
[A/G] &(VAR16D), TM = local VAR32S, then V1DS+=2
|
Operands VAR32x: long variable VAR32x
Axis/Group:
• | An integer 1 to 255 representing an Axis ID |
• | G followed by an integer 1 to 8 representing one of the 8 groups |
• | B for a broadcast to all axes |
dm: data memory operand
TypeMem: memory operand. One of dm (0x1), pm (0x0) or spi (0x2) values
(VAR16x): contents of variable VAR16x, representing a 16-bit address of a variable

Binary code
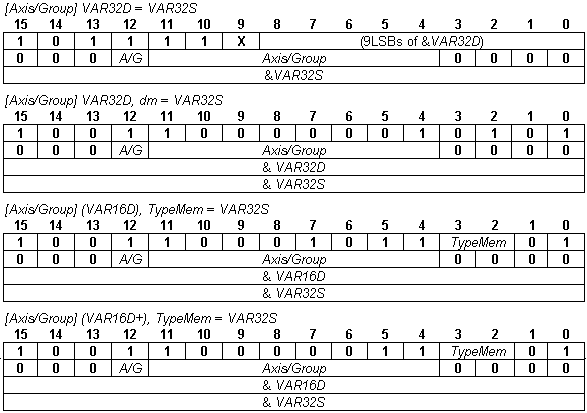
Description | Sends the value of a 32-bit local TML variable to another axis or group of axes. The remote destination can be a 32-bit TML variable or 2 consecutive memory locations with lower address indicated by a 16-bit TML variable (a pointer) from the remote axis/axes. If the pointer variable is followed by a + sign, after the assignment, it is incremented by 2. In the binary code, Axis/Group represents either an Axis ID (if A/G=0) or a Group ID (if A/G = 1). A transmission with Group ID can be: |
• | For all the axes from a single group, if one bit from the 8-bit Group ID is 1 |
• | A broadcast to all the axes, if the Group ID = 0 |
Remark: If the TML variables from the remote axis are user variables, these must be declared in the local axis too. Moreover, for correct operation, these variables must have the same address in both axes, which means that they must be declared on each axis on the same position. Typically, when working with data transfers between axes, it is advisable to establish a block of user variables that may be the source, destination or pointer of data transfers, and to declare these data on all the axes as the first user variables. This way you can be sure that these variables have the same address on all the axes.
The memory location can be of 3 types: RAM for data (dm), RAM for TML programs (pm), EEPROM SPI-connected for TML programs (spi).

One instruction uses a 9-bit short address for the source variable. Bit value X specifies the destination address range:
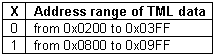
All predefined or user-defined TML data are inside these address ranges, hence these instructions can be used without checking the variables addresses. However, considering future developments, the TML also includes assignment instructions using a full address where the destination address can be any 16-bit value. In this case destination variable is followed by “,dm”.
Execution | Copies a 32-bit value from a local source to a remote destination |
Example1
long VarLoc, VarExt;
...
[15]VarExt = VarLoc;
Before instruction
|
|
After instruction
|
VarLoc on local axis
|
0x1234ABCD
|
|
VarLoc on local axis
|
0x1234ABCD
|
VarExt on axis 15
|
x
|
|
VarExt on axis 15
|
0x1234ABCD
|
Example2
long VarLoc, VarExt;
...
[15]VarExt, dm = VarLoc;
Before instruction
|
|
After instruction
|
VarLoc on local axis
|
0xF0E1A2B3
|
|
VarLoc on local axis
|
0xF0E1A2B3
|
VarExt on axis 15
|
x
|
|
VarExt on axis 15
|
0xF0E1A2B3
|
Example3
long VarLoc;
int pVarExt;
...
[15](pVarExt), dm = VarLoc;
Before instruction
|
|
After instruction
|
VarLoc on local axis
|
0x2233FEDC
|
|
VarLoc on local axis
|
0x2233FEDC
|
pVarExt on axis 15
|
0x1234
|
|
pVarExt on axis 15
|
0x1234
|
At dm address 0x1234 on axis 15
|
x
|
|
At dm address 0x1234 on axis 15
|
0xFEDC
|
At dm address 0x1235 on axis 15
|
x
|
|
At dm address 0x1235 on axis 15
|
0x2233
|
Example4
long VarLoc;
int pVarExt;
...
[15](pVarExt+), dm = VarLoc;
Before instruction
|
|
After instruction
|
VarLoc on local axis
|
0x2233FEDC
|
|
VarLoc on local axis
|
0x2233FEDC
|
pVarExt on axis 15
|
0x1234
|
|
pVarExt on axis 15
|
0x1236
|
At dm address 0x1234 on axis 15
|
x
|
|
At dm address 0x1234 on axis 15
|
0xFEDE
|
At dm address 0x1235 on axis 15
|
x
|
|
At dm address 0x1235 on axis 15
|
0x2233
|
|